VSCode settings for Django
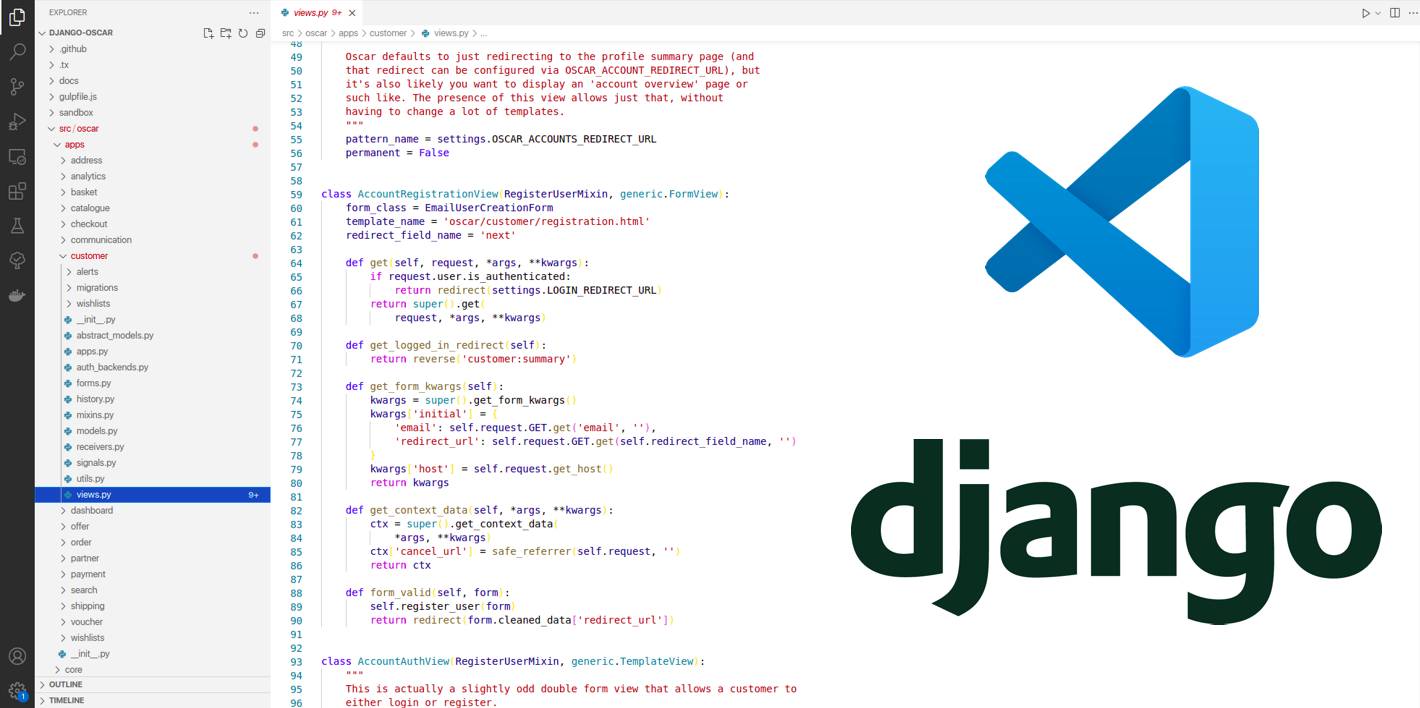
You’ll find VSCode settings for projects that use Django web framework.
How to set up VSCode for Django development.
Straight to the point article about settings, which would enable you to:
- set project’s virtual environment;
- make
flake8
work seamlessly; - launch
runserver
command with Run ▶️ button; - launch
pytest
-tests using buttons from “Tests” tab; - properly sort imports, when saving
python
files; - create a ruler at 79 symbols, so that it’s easier to follow PEP8;
- launch django-tests with button at “Run” tab;
1. Install plugins
For starters you need to install the following plugins:
- Python — usually, it’s pre-installed;
- Cornflakes;
- Djaneiro.
2. Add VSCode settings:
- Create
.vscode
folder in projects directory, if it’s not already there. - Add
launch.json
file in.vscode
folder, with the following contents:
{
"version": "0.2.0",
"configurations": [
{
"name": "Python: Django",
"type": "python",
"request": "launch",
"program": "${workspaceFolder}/manage.py",
"args": [
"runserver"
],
"django": true
},
{
"name": "Python: Django Tests",
"type": "python",
"request": "launch",
"program": "${workspaceFolder}/manage.py",
"args": [
"test",
],
"django": true
},
]
}
With these settings we make it possible to:
- Launch django development server —
runserver
with a click of a button; - Launch django tests —
manage.py test
— with just clicking a button.
Add settings.json
file in .vscode
folder, with the following contents:
{
// path to our virtual environment
// on Windows machines replace «bin» with «Scripts», «python» with «python.exe»
// instead of "/" use "//" or "\"
"python.pythonPath": "<path to your virtual environment>/bin/python",
"python.testing.pytestArgs": [],
"python.testing.unittestEnabled": false,
"python.testing.nosetestsEnabled": false,
"python.testing.pytestEnabled": true,
"python.linting.enabled": true,
"python.linting.pylintEnabled": false,
"python.linting.flake8Enabled": false,
// path to flake8 executable, if it is installed outside your virtual environment
"cornflakes.linter.executablePath": "<path to flake8>",
"cornflakes.linter.run": "onType",
"files.insertFinalNewline": true,
"editor.rulers": [
80,
],
"workbench.editor.enablePreview": false,
"editor.minimap.enabled": false,
"[python]": {
"editor.codeActionsOnSave": {
"source.organizeImports": true
}
}
}
3. Settings for the python project.
If you don’t have setup.cfg
file in your project’s root folder, then add it, if it exists, then just paste the following code into it:
[isort]
balanced_wrapping = false
blocked_extensions=rst,html,js,svg,txt,css,scss,png,snap,tsx
combine_as_imports = true
default_section = THIRDPARTY
force_single_line = false
force_to_top = django
include_trailing_comma = true
known_third_party = django
line_length = 79
lines_between_types=1
multi_line_output = 5
skip=.git,LC_MESSAGES,.pytest-cache
skip_glob=*/migrations/*,*/__pycache__/*
use_parentheses = true
It sets up isort to sort imports according to Django coding style:
Put imports in these groups:
future
,standard library
,third-party libraries
,other Django components
,local Django component
,try/excepts
. Sort lines in each group alphabetically by the full module name. Place all import module statements before from module import objects in each section. Use absolute imports for other Django components and relative imports for local components.On each line, alphabetize the items with the upper case items grouped before the lowercase items.
Break long lines using parentheses and indent continuation lines by 4 spaces. Include a trailing comma after the last import and put the closing parenthesis on its own line.
Use a single blank line between the last import and any module level code, and use two blank lines above the first function or class.
Example from documentation (comments are for explanatory purposes only):
# future
from __future__ import unicode_literals
# standard library
import json
from itertools import chain
# third-party
import bcrypt
# Django
from django.http import Http404
from django.http.response import (
Http404, HttpResponse, HttpResponseNotAllowed, StreamingHttpResponse,
cookie,
)
# local Django
from .models import LogEntry
# try/except
try:
import yaml
except ImportError:
yaml = None
CONSTANT = 'foo'
class Example:
# ...
Hope, you found the article helpful :)